What's A Cache Buster?
To load pages faster, web browsers keep local copies of your site's static assets (JavaScript, CSS, HTML, etc.) so they don't have to download them from your server each time a page is loaded. This is good.
However, after you upload a new version of these files, those old local copies are still used for a while. That's bad. It can break your site in some cases and stops users from seeing your newest changes right away.
CodeKit's cache-buster forces browsers to download a fresh copy of an asset when it has changed.
How Does It Work?
When you turn on the cache-buster, CodeKit scans for <script> and <link> tags that include an external JavaScript file or Stylesheet. It then changes the URL of that external file to include an extra query parameter, like this:
// Before: <script src="file.js" /> // After: <script src="file.js?ckcachebust=0b5a866de46caca8e9" />
This works because browsers will re-download "file.js" if the query parameter has changed since the last time they downloaded the file.
Plan A: MD5 Hash
If CodeKit finds the file referenced by your URL, it will hash the contents of the file and use that hash as the cache-busting query parameter. Since the hash only changes if the file's content does, caches are busted only when they need to be. That keeps your pages loading fast.
Plan B: Timestamp
If CodeKit can NOT find the file in your URL, it will use the current Unix timestamp as the cache-busting query parameter. This is a good fallback, but it means that the cache will always be busted when you upload new files.
Enabling The Cache Buster
Cache-busting is available for every type of file that compiles to HTML (Slim, Markdown, Kit, etc). It is also available for "generic" files and any custom languages that you define.
For One File
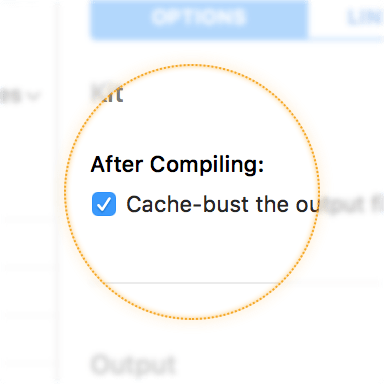
To enable cache-busting for individual files, select a file, then check the "Cache-bust the output file" checkbox.
Note: If you don't see this checkbox, verify that your file's Output Action is set to "Process/Compile".
For All Files Of A Type
Alternately, to enable it for all files of a given type at once, open Project Settings, choose the appropriate language, then check the same box:
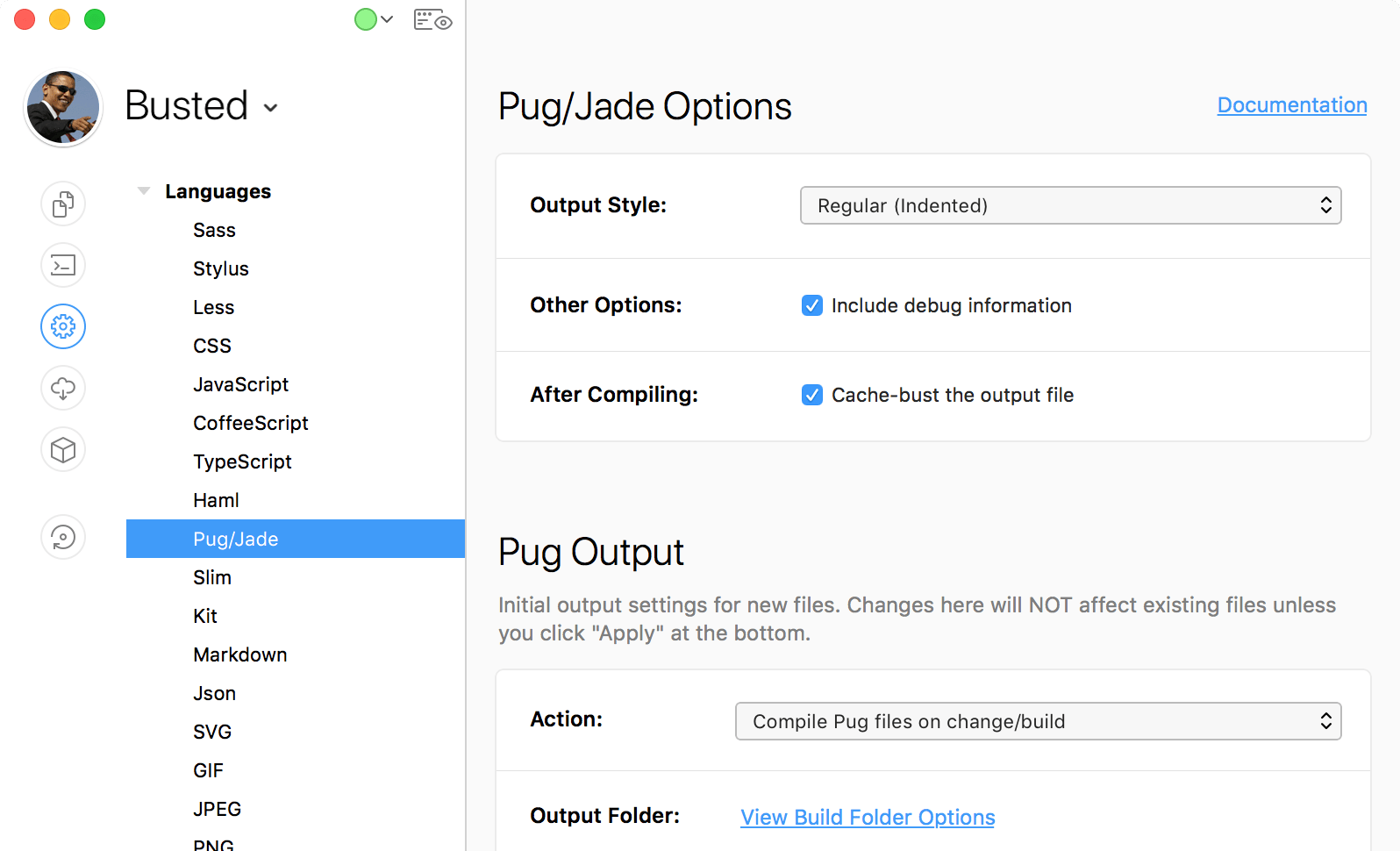
A Few Details
Build Order
During a build, CodeKit will always process JavaScript and Stylesheet files first, before handling page-like files. This ensures that the output files are in place so that the cache-buster can find them and hash them properly.
If you have added a custom Build Step to process certain files before/after others, that order will still be honored. CodeKit will only order the files that you do not explicitly include in any "Process Files" Build Step.
Always Last
The cache-buster is always the last processing step CodeKit performs on a file. If you have set any Hooks to run on your file, those will be executed before cache-busting takes place.
UTF-8
As with all tools in CodeKit, the cache-buster expects your file to use UTF-8 text encoding. After modifying the file, CodeKit will always write it to disk using that encoding.
Implementation
The cache-buster is a custom tool written in C. It is massively fast and should complete virtually instantaneously on any file.
It also handles malformed files gracefully. If a syntax error makes it impossible for CodeKit to understand your <script> or <link> tag, the cache-buster simply skips that tag and continues to the next one it can understand.