What's Rollup?
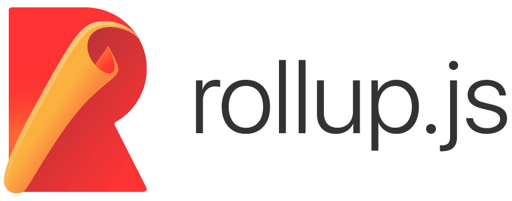
JavaScript now has import statements. Unfortunately, it will be many years before most browsers can understand them natively. Until then, Rollup "bundles" modular JavaScript into a single file that today's browsers can use.
Why Do This?
Because modular JavaScript lets you write cleaner, better-organized code. You can separate things into multiple files, then roll them into a single file for the browser. Also: lots of popular frameworks now ship modular JS that requires bundling.
A Simpler Method
If you don't want to (or can't) use ES6 modules, CodeKit offers another way of combining JavaScript files using simple comments. Click here for details.
Enabling Bundling
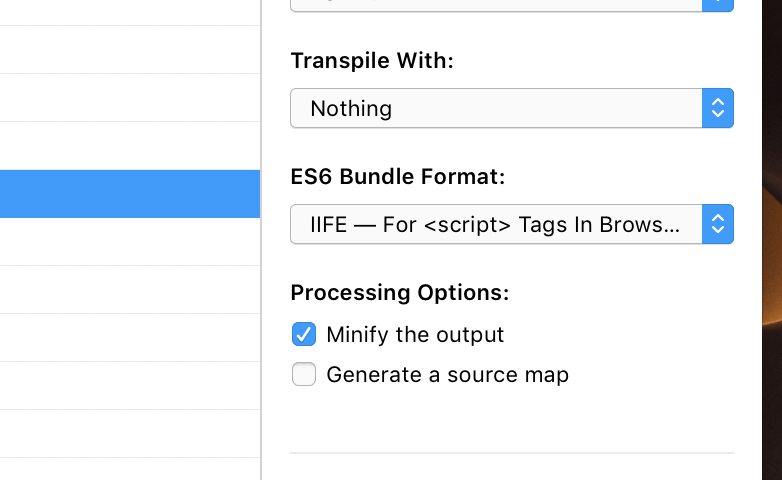
Select a JavaScript file, then choose the type of bundle you want to create from the pop-up button in the File Inspector:
Alternately, you can enable bundling for every JS file at once by opening Project Settings and choosing the JavaScript language.
Bundle Formats
IIFE
"Immediately Invoked Function Expression". In English, that means: "Wrap all my code in a big function, then call the function at the bottom of the file." This is what you want if your script is running in a web browser.
UMD
"Universal Module Definition". This format runs in both web browsers and node.js environments. The output file will be a little bigger, though.
CommonJS
If your script is designed to run in a node.js environment only, this is the format you should choose.
AMD & System
These are available, but they're likely not the right choice unless you're doing some specialty development.
Rollup Options
Open Project Settings and choose 'Rollup' from the 'Tools' category:
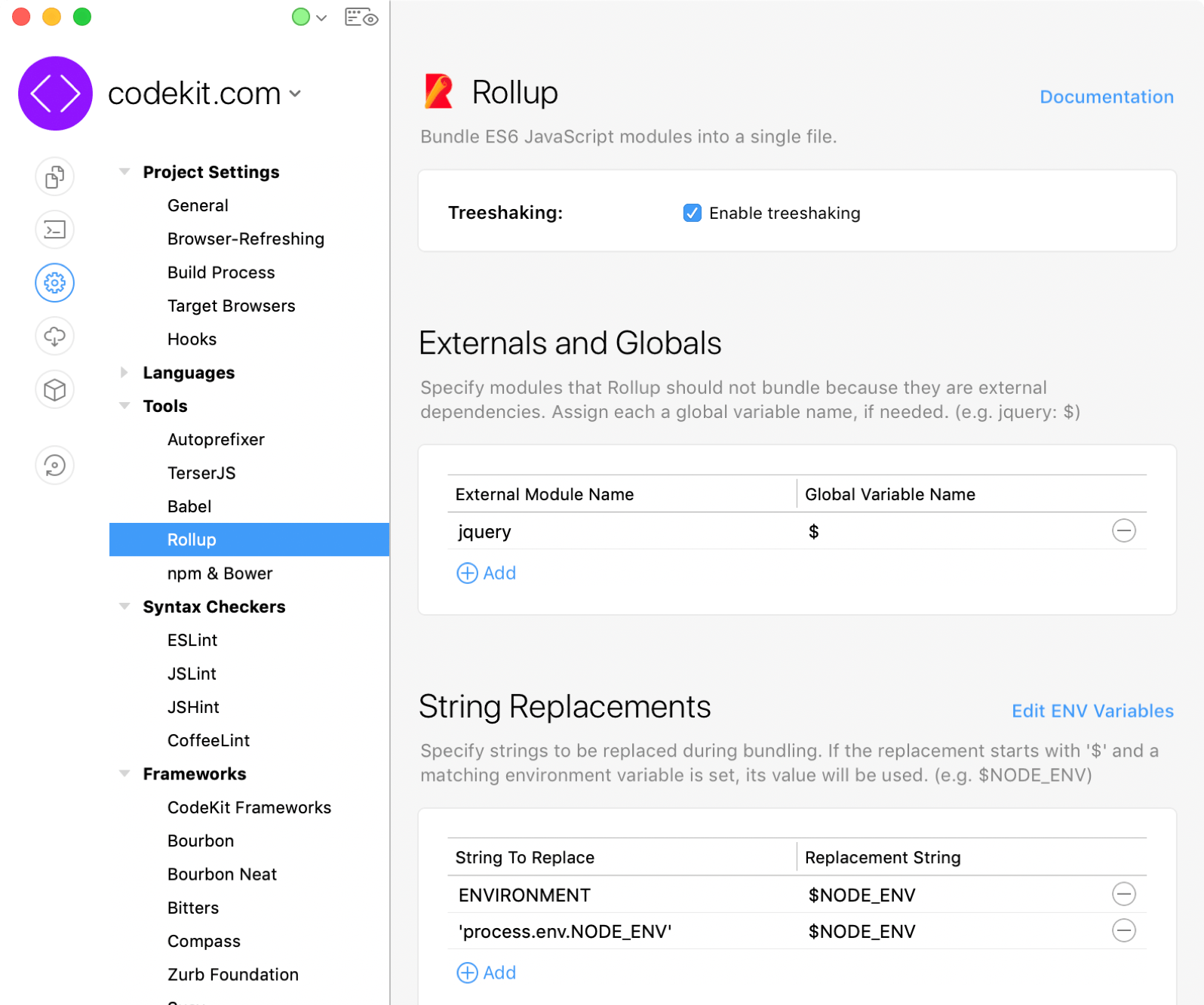
Tree Shaking
To keep files small, Rollup includes only things that are actually used somewhere in the file. If you import a variable or function but don't use it, the import is ignored. If needed, you can disable Tree Shaking.
Externals & Globals
Sometimes, you might import modules that should not be bundled. For example, you might import jQuery, but load it from a CDN. Since jQuery is not installed anywhere in the project, Rollup will throw an error telling you that it can't find jQuery. Resolve that error by telling Rollup that 'jquery' is an external dependency. You can optionally specify a global variable name to which the external dependency should be bound.
String Replacements
Specify strings that should be replaced when Rollup runs. For example, if you import CommonJS modules (node packages), you need to replace the string "process.env.NODE_ENV" or your bundle won't run in a browser, since "process" is not defined in a browser.
If your replacement string starts with a $ and you have defined an Environment Variable with that name, CodeKit will use the value of the environment variable.
Examples
Importing Packages
Suppose you install jQuery in your project using either npm or Bower. In your JavaScript file, you'd write this:
import $ from "jquery" $(document).ready(function { // Do stuff with jQuery. });
As long as the package you're importing is installed via npm or Bower, you don't need to specify a path in your import statement. Just use the module name and CodeKit will find it. Note: packages that don't support ES6 modules can't be imported like this.
Importing Your Own Files
Suppose you have two JS files: main.js and other.js. Here's what "other.js" looks like:
// other.js export var answer = 42; export function sayHello() { console.log('hello'); } export function sayBye() { console.log('bye'); }
Notice the "export" keyword. This is the golden rule of JS bundling:
If you want to IMPORT a thing, first you have to EXPORT the thing.
Now, in main.js we can import like this:
// main.js import {answer, sayHello, sayBye} from "./other.js" sayHello(); // logs "hello" var newAnswer = answer + 10; // 52
Notice the ./ in our import path. This is CRITICAL. It tells Rollup: "the file I want to import is not a package installed by npm or Bower, it's my own file and here is its relative path from the file we're in." If you omit this, your import will fail. The path you provide must always start with ./.
Also notice that we explicitly specified what to import: {answer, sayHello, sayBye}. Those are now available to use in main.js. This is powerful for handling large files: you import only the parts that you need.
Important Notes
Module Names
CodeKit uses the name of the base file as the module name for a bundle, when one is required. If the filename contains characters that are illegal for JS module names, those characters are dropped. If your filename is a protected keyword in JavaScript (e.g. "function"), the module name defaults to "codekitModule".
ES6 Modules + ESLint
ESLint is designed to handle ES6 Modules, but must be told that a file is a module. CodeKit does this automatically by default. You can change that in Project Settings > ESLint by adjusting the "Source Type" option.
Controlling Warnings In Imported Files
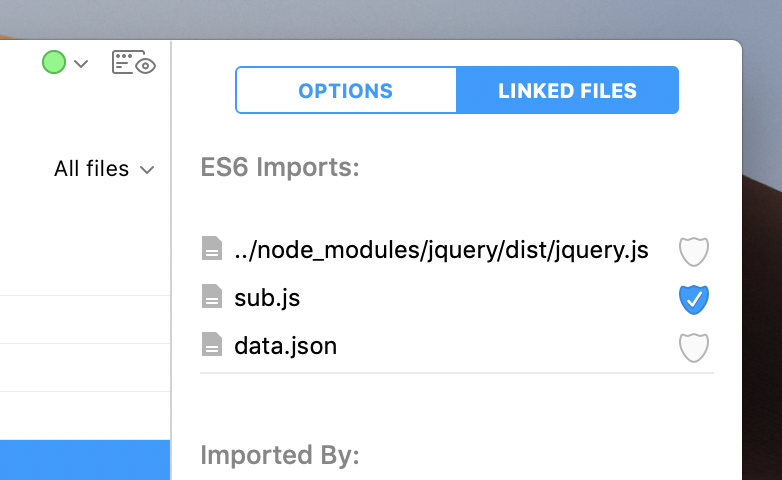
If you don't want to see issues in an imported file, select your base file in CodeKit, then look at the Linked Files pane of the File Inspector:
Uncheck the Shield Checkbox next to the files for which you don't want to see warnings. Note that CodeKit will skip that imported file and any files it may import as well. CodeKit automatically unchecks this shield for any file in a node_modules or bower_components folder.
Pro-Tip: If you add //quiet to the end of your import statement, CodeKit will automatically uncheck the shield:
import {thing} from "./other.js" // quiet // "other.js" will be skipped during syntax-checking
FAQ
Can I Transpile And Bundle Files?
Absolutely. Turn on both options and CodeKit will do the rest.
Why Are There So Many Bundle Formats?
TL;DR—The industry is (slowly) standardizing on the new "import" statement. Your code should use "import" instead of "require" to be futureproof.
The JavaScript language did not have an import statement for a long time. Folks came up with workarounds for that missing functionality. The biggest one was "requireJS" which used a require statement to link files together. Lots of developers came to rely on that, including node.js. When JavaScript finally did add an "import" statement, it used different semantics than "require" and the two systems were not compatible. So we currently live in a world where node.js still uses "require" and web browsers are adopting "import". Eventually, node plans to use "import" as well.